How to integrate your Python App with Slack using Bots
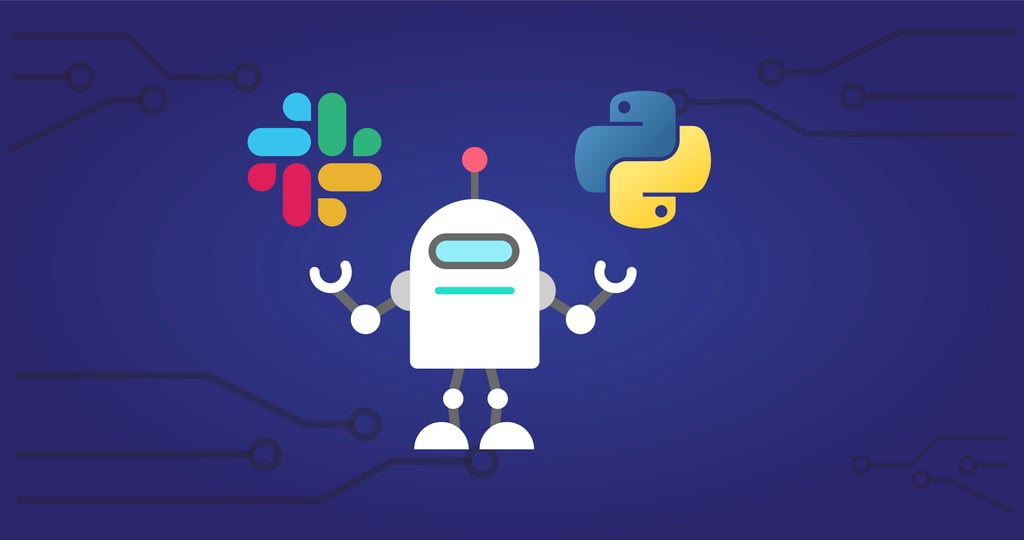
At Swapps, we use Slack daily to communicate with the other members of the work team; from sayting good morning, to notifying a situation of great relevance. Because Slack is an application we use constantly, we have integrated different notifications to this tool; so, for example, in a matter of seconds, we know if a site has been dropped, or if the tests of a pull request have failed.
Slack allows you to create applications that can be integrated into the conversation channels. As I mentioned earlier, these applications can be simple notifications sent when an event occurs in an external application or applications that allow reading and replying to messages or conversations using Bots. A Bot is a type of Slack application designed to interact with users through a conversation. They can be built with events or with Real Time Messaging, RTM.
We are going to create our little Bot, to which we are going to greet and he will answer us. For this example, we will use the subscription to events.
We create Slack’s APP:
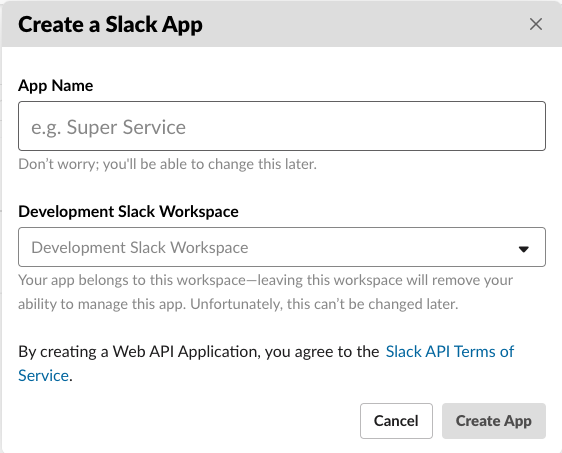
We create the Bot User
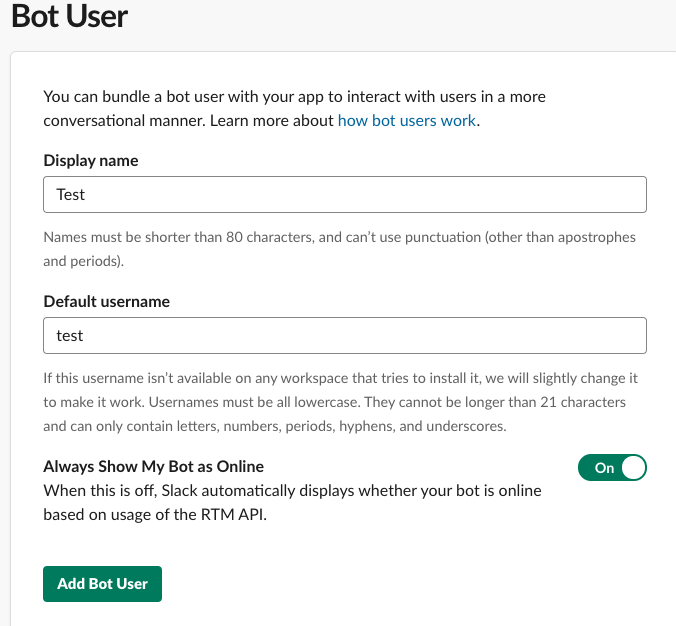
We install the application in the work environment
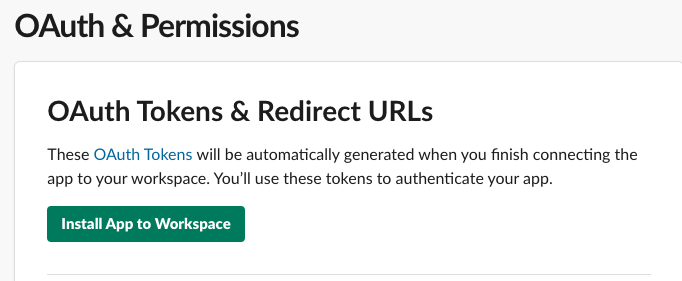
After installation, we copy the Bot User OAuth Access Token and the Verification Token, which is found in the basic information credentials, and save it for later use in our Python application.
Create the resource in our Python Application
Before activating the event subscription, we must create the resource to which Slack will make the requests in our Python App.
Slack requires confirming that the resource works correctly. To this end, Slack sends a request with a JSON similar to the following example and expects, in response, the value of “challenge”; that we must return from our Python app.
{ "token": "Jhj1dZrFaK1ZwHjjRyZWjbDl", "challenge": "3eZbrw1aBm2rZgRNFdxV2595E9CY3gmdALWMmHkvFXO7tYXAYM8P", "type": "url_verification" }
The example app was made using Django, but it can be made using another similar tool like Flask, additionally we use the Slack’s client for Python:
The library is installed with the following command:
Pip install slackclient
To initialize the client we use the token previously added in our App:
from slack import WebClient class SlackBotTest(object): def __init__(self, request): self.Client = WebClient(<SLACK_BOT_USER_TOKEN>) self.slack_message = request.data self.user = None self.channel = None
We must return the same challenge attribute, additionally, it is advisable to validate that the token we receive is the same one we have saved.
if self.slack_message.get("token") != <SLACK_VERIFICATION_TOKEN>: return {"status": HTTP_403} # verification challenge if self.slack_message.get("type") == "url_verification": return self.slack_message.get("challenge")
We activate the event’s subscription
The previous code is returned in a resource that we create “api / slack-events-test”, we enter the URL and with the verified URL we can continue configuring the event subscription. The Python application is developed locally so that Slack can make the request from the outside I have exposed my local instance to public access using ngrok.

In the same configuration, we must choose the event in which the Bot will respond, the Event: message.im, which means that we can interact with it when we mention the bot in the direct channel.
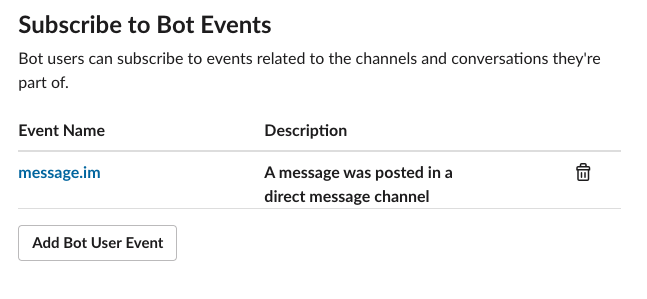

In the end, we save the changes. At this time, we have already configured the Bot using event subscription; we only have to implement the logic so that the Bot salutes.
To the code we have created, we add the logic to get the text that comes from Slack. In case it contains the word “hello”, we proceed to return the response to Slack, with the api_call () method:
from slack import WebClient class SlackBotTest(object): def __init__(self, request): self.Client = WebClient(<SLACK_BOT_USER_TOKEN>) self.slack_message = request.data self.user = None self.channel = None if self.slack_message.get("token") != <SLACK_VERIFICATION_TOKEN>: return {"status": status.HTTP_403_FORBIDDEN} # verification challenge if self.slack_message.get("type") == "url_verification": return self.slack_message.get("challenge") if "event" in self.slack_message: event_message = self.slack_message.get("event") self.user = event_message.get("user") self.channel = event_message.get("channel") if "hello" in event_message.get("text"): self.salute() return {"status": HTTP_200}
In the salute() method we create the response and send it:
def salute(self): bot_text = "Hi <@{}> :wave:".format(self.user) self.api_call(bot_text)
And finally, we make use of the api_call () method of the Slack client, to send the answer:
def api_call(self, bot_text): self.Client.api_call( "chat.postMessage", json={ "channel": self.channel, "text": bot_text, }, ) return {"status": status.HTTP_200_OK}
To try our little Bot, we look for it in our work environment:
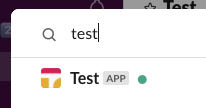
and we write to it:
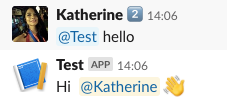
If we want our Bot to be very intelligent and sophisticated we can add natural language processing to the answers. However, using conditionals, we can have a pretty good result. Some ideas to use Slack API can be: integrate an external project to consult important information; receive alerts when a project budget has been exceeded; congratulate the members of your team for their birthday or for their good performance automatically; post a post to different social networks, etc.
As we have seen, building our Bot has been easy, it is quite useful to use the tools that Slack gives us since we can improve the communication of team members, we can automate processes, prevent and act in case of eventualities, the use we can give depends on our imagination and work needs.