How to create an ajax form with drupal 7
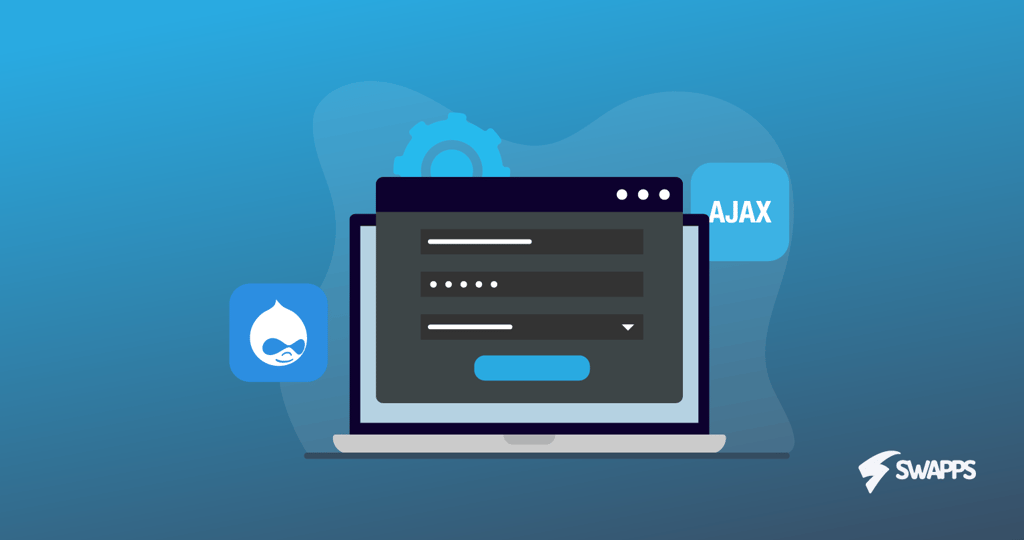
In this tutorial, I am going to explain how to create an ajax form within a block. This is useful when we want to avoid the page reload after submission. We are going to create a small form with one input text and a submit button. After the user clicks the submit button, the input text will hide and will show a message with the previous input submitted.
Let’s create a custom module called my_form:
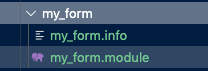
Inside my_form.info, we define our module with the basic information:
name = My Form description = My Ajax form core = 7.x
Inside my_form.module, define the block:
function my_form_block_info() { $blocks = array(); $blocks['my_form'] = array( 'info' => t('my Form'), ); return $blocks; }
After we define these basic settings, activate the module.
In the Block section search the new block:
admin/structure/block
In our current theme, select the section where we want to show our block, in this example, it would be content
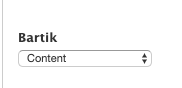
I only want to show this form on a specific page:
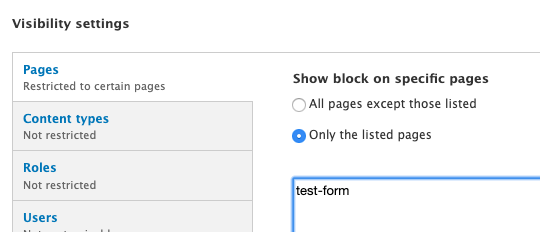
After we save it, we can see our block like this:

We must create a basic page called test-form, right now the page will be empty because we don’t have the block view defined yet.
Let’s define the view of our block:
function my_form_block_view($delta='') { $block = array(); switch($delta) { case 'my_form' : $content = drupal_get_form("my_custom_form"); $block['content'] = array( "form"=>$content); break; } return $block; }
As we can see, we called the function drupal_get_form, this will look for the function my_custom_form and will render it inside the block.
Now, let’s create the function my_custom_form:
function my_custom_form($form, &$form_state){ $form['box-container'] = array( '#prefix' => '<div id="box">', ); $form['name'] = array( '#suffix' => '</div>', '#type' => 'textfield', '#required' => true, '#attributes' => array('id' => 'name', 'placeholder'=> 'Name'), ); $form['submit'] = array( '#type' => 'submit', '#value' => t("Submit"), '#ajax' => array( 'callback' => 'ajax_submit_callback', 'wrapper' => 'box', ), ); return $form; }
Let’s explain how this actually works:
- The first item in our form is called box-container, we use this item to contain the section of the input text, the only attribute that it has is:
- Prefix: Text or markup to include before the form element.
- Then we have defined an input text called name, and the attributes:
- Suffix: Text or markup to include after the form element
- Type textfield: Format a single-line text field.
- Required: Indicates whether or not the element is required.
- Attributes: Additional HTML attributes, such as ‘class’ can be set using this mechanism.
- The last item is the submit button:
- Type Submit: Format a form submit button.
- Value: Used to set values that cannot be edited by the user.
- Ajax[‘callback’]: Specifies the name of a callback function which will be called during an AJAX call. It can return HTML, a renderable array, or an array of AJAX Commands. This callback function is given the $form and $form_state parameters, allowing it to produce a result, which it returns for rendering.
- Ajax[wrapper]: This property defines the HTML id attribute of an element on the page which will be replaced by the HTML returned by the #ajax[‘callback’] function
When we set the ajax callback in the submit button, a class name ajax-processed is defined and that avoids the page reload when we click the button. In addition to that, it establishes a dynamic ID to the submit button. it is important to not set manually a different ID to this button (as we did with the input name) because that will make the ajax form fail.
Now we define the method ajax_submit_callback, this will return the new html content that will override the container with the ID box defined in ajax[‘wrapper’]. In this function we obtain the box-container item and set the new content using the attribute markup:
function ajax_submit_callback($form, $form_state) { $element = $form['box-container']; $element['#markup'] = '<h6>hello '.$form_state['values']['name'].'!</h6>'; return $element; }
Finally, we can see our form:
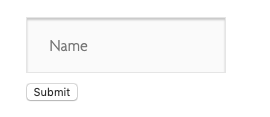
After submit, we should see the new content:
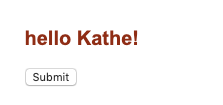
We have learned how to create an ajax form and can be very useful in many scenarios, improving the user experience of your site. We also have the possibility to render this form in any place that a block allows. For example, if you want to subscribe or register users. You can also make some requests to an API in the background or check data against the database and return if a user already exists without reloading the page. Now you are ready to change your traditional forms into ajax forms.