How To Automate The Workflow In Frontend
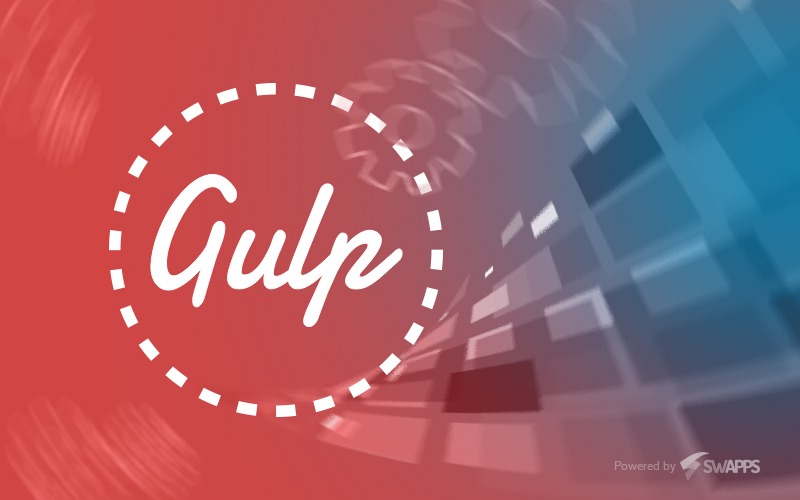
The idea of this post is to learn how you can automate your job in the front-end area, because it doesn’t include just to translate a design onto the web, but also how you can save time and work when building your page, e.g. compiling JavaScript and CSS files (SASS, LESS, Stylus, etc) and reloading the browser automatically when you save any of these files. All of this is possible using a toolkit called Gulp.js, used for automating time-consuming tasks in your development workflow; besides is an open-source JavaScript project.
With Gulp.js you can:
- Minify your HTML, CSS and JS files.
- Compile CSS preprocessors like SASS or LESS.
- Reload the browser automatically whenever you save a file.
- Optimize all assets (CSS, JS, fonts and images).
You will also learn how to chain together different tasks into simple commands that are easy to understand and execute. Next, I’m going to show how to install Gulp and configure it.
Installation
Step One
We must have installed the most important requirement, Node.js. If you don’t have it installed, you can go to http://nodejs.org and download the latest stable version. The Node installer will guide you through the process.
Step Two
Now let’s go to install Gulp, to do it just execute this command line in your terminal:
npm install --global gulp-cli
That way you will install Gulp on your computer, to install it into your dependencies we must go to your project directory on your console and run this command:
npm install --save-dev gulp
Step Three
Is necessary to create a configuration file called gulpfile.js in your project folder, there is where we’re going write all the tasks, including to compile SASS, minify CSS, compile and minify JavaScript files, minify HTML, among others. Now we will add some basic code to get started:
var gulp = require('gulp'); gulp.task('default', function() { // place code for your default task here });
On three simple steps we installed Gulp.js, now we’ll configure a project to run simple tasks below.
Using Sass
First we must install the SASS plugin, that’s why we’re going to run:
npm install gulp-sass --save-dev
Next, we must call the plugin installed on gulpfile.js, so we will do it:
var gulp = require('gulp') var sass = require('gulp-sass') gulp.task('sass',function(){ gulp.src('styles/main.scss') .pipe(sass({style:'expanded'})).on('error',gutil.log) .pipe(_.concat('style.css')) .pipe(gulp.dest('assets')) });
This task will compile a SASS file and convert it into a CSS file. THe source for compiling is “styles/main.scss” and will be saved as “assets/style.css”. To execute this task, please run this command:
gulp sass
Minify css
Now that you can compile your SASS code into CSS it can be used in your HTML, but to improve performance and page load we can minify it. To achieve this, we will install gulp-clean-css:
npm install gulp-clean-css --save-dev
var gulp = require('gulp') var cleanCSS = require('gulp-clean-css'); gulp.task('minify-css', function() { return gulp.src('assets/style.css') .pipe(cleanCSS()) .pipe(_.rename({ extname: '.min.css'})) .pipe(gulp.dest('assets')); });
This task will minify a CSS file, in this case “assets/style.css” and it will add a “.min.css” extension (that means it is a minified file) and it will be saved as “assets/style.min.css”. To execute this task, please run this command:
gulp minify-css
Using JS
To compile and minify JavaScript we will need to install some plugins, gulp-uglify and gulp-contact, so we will install them:
npm install --save-dev gulp-uglify npm install --save-dev gulp-concat
At this point, you can use them this way:
var _ = require('gulp-load-plugins')({lazy: false}); var uglify=require('gulp-uglify'),concat=require('gulp-concat'); gulp.task('build-js', function() { gulp.src('scripts/*.js') .pipe(uglify()) .pipe(concat('script.js')) .pipe(gulp.dest('assets')) });
This task will compile and minify the JS files found in “scripts/” and they will be saved into a single file, “assets/script.js”. To execute this task, please run this command:
gulp build-js
Watching Files
If you want your browser to reload itself when some files are changed, first you need to install Browsersync:
npm install browser-sync --save-dev
var browserSync = require('browser-sync').create() gulp.task('watch', function() { gulp.watch('scripts/*.js', ['build-js']).on('change', browserSync.reload) gulp.watch('styles/*.scss', ['sass']).on('change', browserSync.reload) gulp.watch( 'styles/*.css', ['minify-css']).on('change', browserSync.reload) });
This task is the most useful of all because it merges all tasks in only one command, with this task you can compile and minify SASS, CSS and JS at the same time, and the best part is that you don’t need to run this task everytime, because once it’s executed it will be running in the console. This command will reload your browser when you make any changes and save any file of the mentioned. To execute this command use:
gulp watch
If you want all the tasks here below we are going to leave them, with this you can make your job easier, as it will speed up your work and can save time in your work as a frontend developer. We hope this post can help you to automate all these tasks that can be quite boring.
var gulp = require('gulp') var sass = require('gulp-sass') var cleanCSS = require('gulp-clean-css') var _ = require('gulp-load-plugins')({lazy: false}); var uglify=require('gulp-uglify'),concat=require('gulp-concat'); var browserSync = require('browser-sync').create() gulp.task('sass',function(){ gulp.src('styles/main.scss') .pipe(sass({style:'expanded'})).on('error',gutil.log) .pipe(_.concat('style.css')) .pipe(gulp.dest('assets')) }); gulp.task('minify-css', function() {   return gulp.src('assets/style.css')   .pipe(cleanCSS())   .pipe(_.rename({ extname: '.min.css'}))   .pipe(gulp.dest('assets')); }); gulp.task('build-js', function() {   gulp.src('scripts/*.js')   .pipe(uglify())   .pipe(concat('script.js'))   .pipe(gulp.dest('assets')) }); gulp.task('watch', function() {   gulp.watch('scripts/*.js', ['build-js']).on('change', browserSync.reload)   gulp.watch('styles/*.scss', ['sass']).on('change', browserSync.reload)   gulp.watch( 'styles/*.css', ['minify-css']).on('change', browserSync.reload) });